File的常用方法
创建:
createNewFile()在指定位置创建一个空文件,成功就返回true,如果已存在就不创建,然后返回false。
mkdir() 在指定位置创建一个单级文件夹。
mkdirs() 在指定位置创建一个多级文件夹。
renameTo(File dest)如果目标文件与源文件是在同一个路径下,那么renameTo的作用是重命名, 如果目标文件与源文件不是在同一个路径下,那么renameTo的作用就是剪切,而且还不能操作文件夹。
删除:
delete() 删除文件或者一个空文件夹,不能删除非空文件夹,马上删除文件,返回一个布尔值。
deleteOnExit()jvm退出时删除文件或者文件夹,用于删除临时文件,无返回值。
判断:
exists() 文件或文件夹是否存在。
isFile() 是否是一个文件,如果不存在,则始终为false。
isDirectory() 是否是一个目录,如果不存在,则始终为false。
isHidden() 是否是一个隐藏的文件或是否是隐藏的目录。
isAbsolute() 测试此抽象路径名是否为绝对路径名。
获取:
getName() 获取文件或文件夹的名称,不包含上级路径。
getAbsolutePath()获取文件的绝对路径,与文件是否存在没关系
length() 获取文件的大小(字节数),如果文件不存在则返回0L,如果是文件夹也返回0L。
getParent() 返回此抽象路径名父目录的路径名字符串;如果此路径名没有指定父目录,则返回null。
lastModified()获取最后一次被修改的时间。
文件夹相关:
static File[] listRoots()列出所有的根目录(Window中就是所有系统的盘符)
list() 返回目录下的文件或者目录名,包含隐藏文件。对于文件这样操作会返回null。
listFiles() 返回目录下的文件或者目录对象(File类实例),包含隐藏文件。对于文件这样操作会返回null。
list(FilenameFilter filter)返回指定当前目录中符合过滤条件的子文件或子目录。对于文件这样操作会返回null。
listFiles(FilenameFilter filter)返回指定当前目录中符合过滤条件的子文件或子目录。对于文件这样操作会返回null。
基于spring的文件上传
ajax
文件上传,需要使用formData封装参数
(function($){
$("#upload").on("click", function () {
var s = $('#file')[0].files[0];
var formData = new FormData();
formData.append("file_data", s);
formData.append("type", "1");
$.ajax({
url: "SpringMVC/fileUpload",
type: 'POST',
cache: false,
data: formData,
processData: false,
contentType: false,
success: function (result) {},
error: function (err) {}
});
})
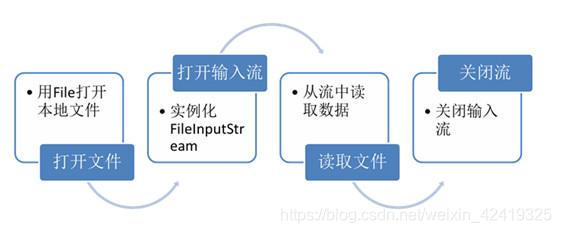
与之对应的java
首先使用MultipartFile 接收参数
@RequestParam(“file”) MultipartFile file
InputStream iStream= null;
try{
iStream = file.getInputStream(); //获取输入流
}
将File转化为MultipartFile
File file = new File("D:\\Desktop\\sql.txt");
FileInputStream input = new FileInputStream(file);
MultipartFile multipartFile = new MockMultipartFile("file", file.getName(), "text/plain", IOUtils.toByteArray(input));
java实现文件上传(使用file实现)
public static String uploadImage(MultipartFile file, String path) throws IOException {
String name = file.getOriginalFilename() ;
String suffixName = name.substring(name.lastIndexOf(".")) ;
Date date = new Date() ;
String hash = DateUtil.toStr(date.getTime());//给照片自定义一个名字,用时间做名称,不会重复
String fileName = hash + suffixName ;
File tempFile = new File(path, fileName) ;
//判断文件夹是否存在,不存在则创建
if(!tempFile.getParentFile().exists()) {
tempFile.getParentFile().mkdirs() ;
}
//在指定位置创建一个空的文件
tempFile.createNewFile() ;
//将file文件转移给tempFile
file.transferTo(tempFile);
return tempFile.getName() ;
}
java实现文件上传(使用输入输出实现)
File file = new File("D:\\Desktop\\sql.txt");
FileInputStream input = new FileInputStream(file);
MultipartFile multipartFile = new MockMultipartFile("file", file.getName(), "text/plain", IOUtils.toByteArray(input));
InputStream iStream = multipartFile.getInputStream();
//创建一个空的文件
FileOutputStream fStream=new FileOutputStream("D:\\Desktop\\test\\sql.txt");
//将iStream文件转移给tempFile
IOUtils.copy(iStream, fStream);
fStream.close();
文件删除
public static boolean deleteImage(String pathName,String fileName){
boolean delete = false;
File folder = new File(pathName);
File[] files = folder.listFiles();
for(File file:files){
if(file.getName().equals(fileName)){
delete = file.delete();
}
}
return delete;
}
基于spring下载文件
public void file(HttpServletResponse response,HttpServletRequest request
//设置响应头,告诉浏览器以下载的方式打来文件,设置中文编码,如果不设置会出现乱码
response.setHeader("content-disposition", "attachment;filename="+URLEncoder.encode(titleName, "UTF-8"));
response.getOutputStream().write(download);
response.getOutputStream().close();
将文件上传至服务器
FastDFSUtil 文件中
name="+URLEncoder.encode(titleName, “UTF-8”));
response.getOutputStream().write(download);
response.getOutputStream().close();
将文件上传至服务器工具类
@Component
public class FastDFSUtil {
private TrackerClient trackerClient;
private TrackerServer trackerServer;
private StorageClient storageClient;
static {
ClientGlobal.setG_connect_timeout(0);
ClientGlobal.setG_network_timeout(0);
ClientGlobal.setG_anti_steal_token(false);
ClientGlobal.setG_charset("UTF-8");
}
public FastDFSUtil() throws Exception {
InetSocketAddress[] tracker_servers = getFastDFSInfo();
TrackerGroup tg = new TrackerGroup(tracker_servers);
this.trackerClient = new TrackerClient(tg);
this.trackerServer = this.trackerClient.getConnection();
this.storageClient = new StorageClient(this.trackerServer, (StorageServer) null);
ProtoCommon.activeTest(this.trackerServer.getSocket());
}
public InetSocketAddress[] getFastDFSInfo() throws Exception {
NacosUtil nacosUtil = new NacosUtil();
String naCosValue = nacosUtil.getNaCosValue("config.properties","DEFAULT_GROUP","fastdfs.hostports");
String [] hostport = naCosValue.split(",");
InetSocketAddress[] tracker_servers = new InetSocketAddress[hostport.length];
for(int i =0;i<hostport.length;i++){
String node = hostport[i];
String[] ipport = node.split(":");
String nodeHost =ipport[0];
int nodePort =Integer.parseInt(ipport[1]);
tracker_servers[i] = new InetSocketAddress(nodeHost, nodePort);
}
return tracker_servers;
}
/**
* 上传文件方法
* <p>Title: uploadFile</p>
* <p>Description: </p>
*
* @param fileName 文件全路径
* @param extName 文件扩展名,不包含(.)
* @param metas 文件扩展信息
* @return
* @throws Exception
*/
public String[] uploadFile(String fileName, String extName, NameValuePair[] metas) throws Exception {
return storageClient.upload_file(fileName, extName, metas);
}
public String[] uploadFile(String fileName) throws Exception {
return uploadFile(fileName, null, null);
}
public String[] uploadFile(String fileName, String extName) throws Exception {
return uploadFile(fileName, extName, null);
}
/**
* 上传文件方法
* <p>Title: uploadFile</p>
* <p>Description: </p>
*
* @param fileContent 文件的内容,字节数组
* @param extName 文件扩展名
* @param metas 文件扩展信息
* @return
* @throws Exception
*/
public String[] uploadFile(byte[] fileContent, String extName, NameValuePair[] metas) throws Exception {
return storageClient.upload_file(fileContent, extName, metas);
}
public String[] uploadFile(byte[] fileContent) throws Exception {
return uploadFile(fileContent, null, null);
}
public Map<String ,Object> uploadFile(byte[] fileContent, String extName) throws Exception {
Map<String ,Object> result = new HashMap<>();
String [] info = uploadFile(fileContent, extName, null);
String group = info[0];
String objectid = info[1];
long size = storageClient.get_file_info(group, objectid).getFileSize();
result.put("EXT", extName);//文件扩展名
result.put("STORAGE_TYPE","FDFS");//文件类型
result.put("FDFS_GROUP", group);
result.put("FDFS_NAME", objectid);//文件存储对象ID
result.put("FDFS_SIZE", size);//文件大小
return result;
}
/**
* 文件下载
*
* @param group_name
* @param remote_filename
* @param savePath 本地保存地址
*/
public boolean download(String group_name, String remote_filename, String savePath) throws IOException, MyException {
byte[] bytes = storageClient.download_file(group_name,remote_filename);
String suffixName = remote_filename.substring(remote_filename.lastIndexOf("."));
Date date = new Date() ;
String hash = toStr(date.getTime());//给文件自定义一个名字,用时间做名称,不会重复
if (bytes == null && "".equals(suffixName)) {
return false;
}
String fileName = hash + suffixName;
File tempFile = new File(savePath,fileName) ;
//判断文件夹是否存在,不存在则创建
if(!tempFile.getParentFile().exists()) {
tempFile.getParentFile().mkdirs() ;
}
tempFile.createNewFile() ;
OutputStream out=new FileOutputStream(tempFile);
IOUtils.write(bytes,out);
IOUtils.closeQuietly(out);
return true;
}
/**
* 文件下载 获取二进制文件
*
* @param group_name
* @param remote_filename
* @return
* @throws IOException
* @throws MyException
*/
public byte[] download(String group_name, String remote_filename) throws IOException, MyException {
return storageClient.download_file(group_name,remote_filename);
}
/**
* 文件删除
*
* @param group_name
* @param remote_filename
* @return
*/
public Boolean deleteFile(String group_name, String remote_filename) {
try {
int i = storageClient.delete_file(group_name,remote_filename);
return i == 0;
} catch (Exception e) {
e.printStackTrace();
return false;
}
}
/**
* 获取文件信息
*
* @param group_name
* @param remote_filename
* @return
*/
public Map<String,Object> getFileInfo(String group_name, String remote_filename) {
try {
Map<String,Object> map = new HashMap<>();
FileInfo fileInfo = storageClient.get_file_info(group_name,remote_filename);
if (fileInfo == null) {
return null;
}
String sourceIpAddr = fileInfo.getSourceIpAddr();//文件IP地址
long fileSize = fileInfo.getFileSize();//文件大小
Date createTimestamp = fileInfo.getCreateTimestamp();//文件创建时间
long crc32 = fileInfo.getCrc32();//错误码
map.put("fileIpAddress",sourceIpAddr);
map.put("fileSize",fileSize);
map.put("fileCreationTime",createTimestamp);
return map;
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
public static String toStr(long time) {
SimpleDateFormat sFormat=new SimpleDateFormat("yyyyMMDDHHmmSS");
String format = sFormat.format(time);
return format;
}
}
本站文章版权归原作者及原出处所有 。内容为作者个人观点, 并不代表本站赞同其观点和对其真实性负责,本站只提供参考并不构成任何投资及应用建议。本站是一个个人学习交流的平台,网站上部分文章为转载,并不用于任何商业目的,我们已经尽可能的对作者和来源进行了通告,但是能力有限或疏忽,造成漏登,请及时联系我们,我们将根据著作权人的要求,立即更正或者删除有关内容。本站拥有对此声明的最终解释权。